Build Design Systems With Penpot Components
Penpot's new component system for building scalable design systems, emphasizing designer-developer collaboration.
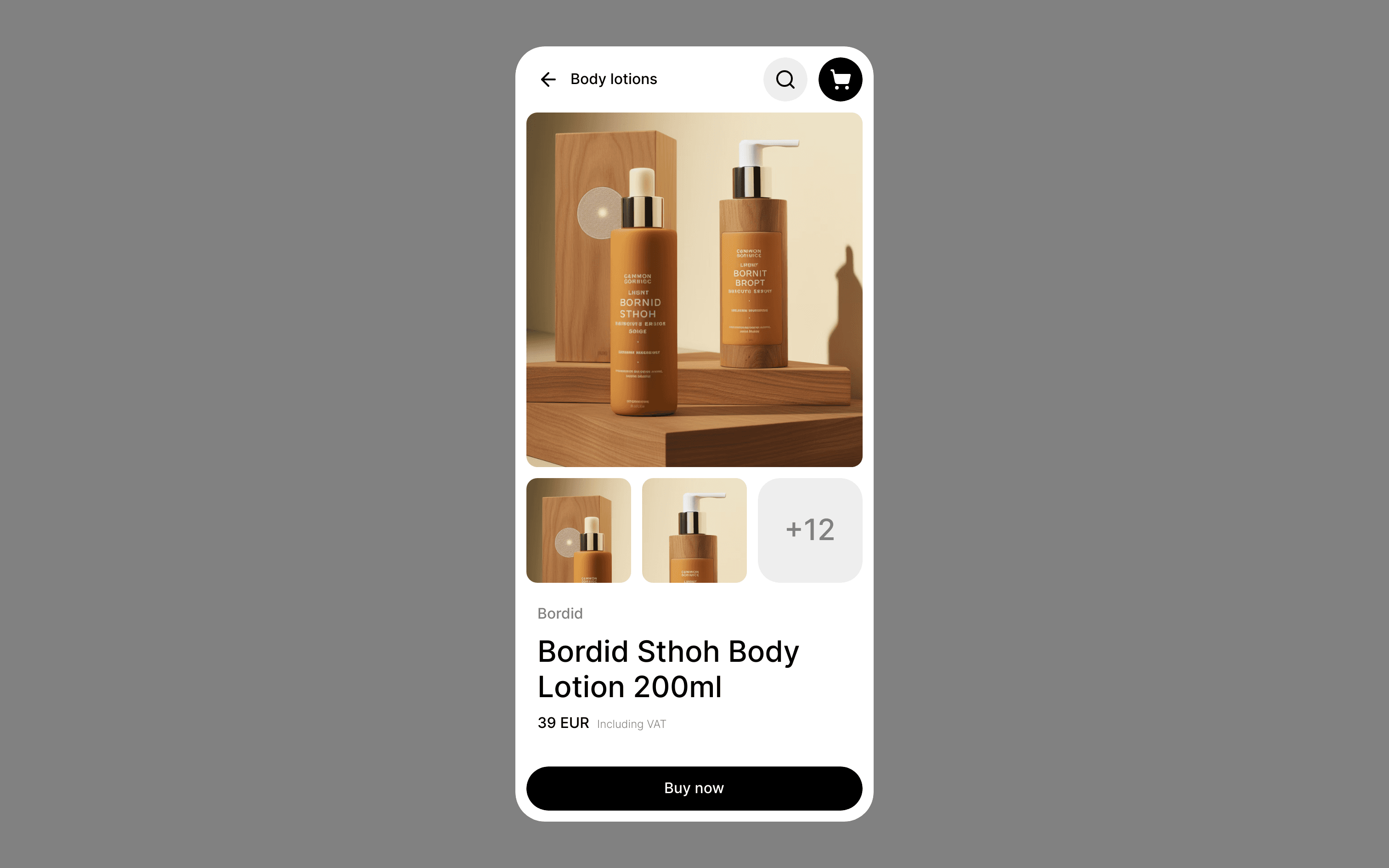
medium bookmark / Raindrop.io |
If you work even remotely near the web dev industry, you’re 100% sure to hear these three infamous letters: API.
They get thrown around by n00bs and experts alike:
“Why do I have to make an API call? Does it have a number?”
“A simple call to this third party API will do the trick here.”
“We’ll build you a RESTful API; making sure your new system plays well with other services.”
While APIs may first seem intimidating, they can literally change your life as a developer.
Why? Well, learning how to use APIs properly can simplify, accelerate, and strengthen your development workflow. Building or integrating APIs brings a fair share of benefits for both clients and yourself.
As developers, you often hear the “don’t re-invent the wheel” advice:
Jokes aside, APIs play a significant role in you not re-inventing the wheel. At Snipcart, we firmly believe that understanding the basics of APIs is a crucial skill for modern web developers. In this post, we’ll help you do just that. We’ll cover:
Let’s dive into it, shall we?
Level up your dev skills with APIs
One of the first, biggest milestones in my programming career was understanding APIs properly.
I still use them every single day.
Before stepping into the nitty-gritty, let me first convince you that understanding APIs is well worth your time.
Because learning to use APIs dramatically increases your development effectiveness.
First, it allows you to leverage pre-existing logic or parts you don’t have to write yourself. Some stuff you might just not be able to code yourself even! So to save yourself precious time, it’s essential, as a developer, to have an idea of what the API landscape looks like.
Second, many development problems you’ll encounter have already been solved by someone before you. Whatever shape these existing solutions take (FaaS, libraries, web services, SDK, content APIs, etc.), you’ll most likely need an API to interact with them.
Well, the official, daunting definition goes like this:
In computer programming, an application programming interface (API) is a set of subroutine definitions, protocols, and tools for building application software. In general terms, it is a set of clearly defined methods of communication between various software components. A good API makes it easier to develop a computer program by providing all the building blocks, which are then put together by the programmer.
A bit heavy, huh? Let’s take the academic prose down a notch. Here’s a friendlier, home-cooked API definition:
Simply put, an API declares an interface for you to interact with its logic without you having to know what happens under the hood. This definition can be applied to whatever language, protocol, or environment you’re in—as long as it happens on the programmatic level (more on that below).
To shed an even better light on APIs, let’s list what they are NOT:
An API is not necessarily an external service. For instance, you can include libraries directly in your solution OR use them through an API.
An API is not just an interface. It’s both the specification/format and the implementation.
An API is not a GUI (graphical user interface). It doesn’t do interactions on the graphical level; it solely operates on the programmatic layer, either through a programming language or a communication protocol.
All APIs aren’t created equal.
Even though they mostly share the same goal, some achieve it way better than others. Since this is to be a smooth intro, I won’t dive into what makes an API better than others. However, keep in mind that people have really different approaches to building APIs. If that subject interests you, Google around “API design patterns” & “API paradigms.” Or just start with this neat entry-level article. 🙂
The goal of APIs is to make your life as a developer easier. How do they do that? By aggregating features/functions set together and exposing these functionalities through endpoints (typically URL patterns used to communicate with the API). These endpoints are the only way you can interact with any API. Each endpoint will have a specified format for both its requests and responses—you usually find this format in the API’s documentation.
Endpoints can either be simple functions or composed of many functions which call other APIs and so on. The only crucial point here is that the underlying logic of those functions is entirely abstracted. You don’t need any knowledge of what’s happening inside them to use them. As long as you use the proper format you will be able to consume them, which is a fancy way of saying using parts of them from your application.
Bottom line, an API is just like any interface: the light switch will turn on the light, whether you know how electric currents function or not (as a young adult I also learned you need to pay the electricity bill to make it work, but that’s a different story.)
Typical API call
I don’t want to overextend conceptual explanations too much here. So let’s analyze a really straightforward API to dumb this down and see how it works in a real-life scenario. We’ll take the native JavaScript Math object to do so (open documentation here).
You can see in the docs that each function of the object describes what the input format should be (number, array of numbers, etc.) and describes the format of the output. Notice, however, how nothing is mentioned regarding the logic to run these functions. For instance, if you fire up your dev console and type Math.sqrt
(without executing the function) you get something like ƒ sqrt() { [native code] }
. That’s the spirit of an API: whether you’re calling one of your Operating System API or a web-based API, these principles will stay.
There’s a ton of stuff to be done with popular API providers out there. The Google Maps API, for instance, is often used to build better user experiences with data based on real-time mapping and traffic signals. The Twitter API, another big one, can be used to filter and display targeted Tweets in real-time.
Now, if you follow our blog, I suspect you’re more interested in web-based APIs than anything else. So let’s dive into specific use cases. Note that we’ll be using Postman in our examples. Of course, this Rest Client is bound to a web environment, but such tools usually exist in other environments also. It’s worth having a look around the tools provided for a given environment before starting to play with it; can save you lots of time.
If you want to follow along with us, you’ll need to download the Postman client.
Our first example is going to be quite simple, but still more interesting than the Math one.
We will be using the Dog API! Not only is it a funny API, but it also doesn’t require any authentication. Plus, it’s a HTTP REST API, which means it’s a web-based API. Since it’s bound to this environment, it requires us to conform to some of the protocol specificity: in this case HTTP Verbs (GET, PUT, POST, DELETE, etc.). For our example, we’ll keep things simple and only use the GET verb. The API doesn’t allow support anything else anyway. Most public APIs only allow you to consume data and not post any, thus the GET verb.
Let’s fire up Postman and see how its UI looks.
My UI uses the dark theme, so it might slightly differ from yours.
There might be a lot of information to digest at first here, so let’s just start with the VERB and URL.
The default verb should be GET so you can keep it this way and enter the following URL:
https://dog.ceo/api/breeds/list/all
Hit “Send” and bang! You should receive a response with the appropriate data. Voilà! You’ve just made your first API call. Now, for this example, we won’t be using any of the data received. But you get the idea: you could show different dog breeds to your user and display an image of a given breed if they click on it.
You can play with the different routes in their documentation to familiarize yourself with the environment.
Cool fact: this little project is also open source, so you can have a look under the hood here.
You should now better understand how different pieces work together when it comes to APIs. So let’s do a more complex tutorial that includes authentication. I’m going to use our own application’s API here.
Here we’ll only use code to interact with Snipcart’s API. You can close Postman, but keep it handy; it’s good to use such a tool when starting out with an API.
The goal will be to create a simple CLI tool to create 1-time usage discounts for shoppers. These discounts will be generated via API, and all this will be happening entirely on your computer.
Create a new folder for this project, use npm init
in the folder and boot up your text editor. Now create a index.js
file, then open the package.json
file. In it, add the following lines in the top level object:
"bin": {
"discounts": "./index.js"
}
We will also need the commander package to parse inputs from the client. So, run npm install --save commander
in the current folder. We will also use a small lib to create IDs; you can install it with npm install --save shortid
. While we’re at it lets also run npm install --save request
lib to make it easier for us to make HTTP calls. Hop back in your index.js
file and paste this code:
#!/usr/bin/env node
var program = require('commander');
var shortid = require('shortid');
var request = require('request');
function CreateDiscount(){
var discount = {
name: '20% OFF',
trigger: 'Code',
type: 'Rate',
rate: 20,
maxNumberOfUsages: 1,
code: shortid.generate()
}
request({
url: "http://app.snipcart.com/api/discounts",
auth: {
'user': 'YOUR_API_KEY'
},
method: "POST",
json: true,
body: discount
}, function (error, response, body){
console.log(body.code);
});
}
program
.arguments('<number>')
.action(function(number) {
for(var i = parseFloat(number); i > 0; i--){
CreateDiscount();
}
})
.parse(process.argv);
Let’s just focus on the request
function here. This is where we make the external API call to Snipcart. You can see we pass the method: "POST"
to the method. This is because we want to post data to Snipcart’s API. By specifying this method, the API will map the action correctly so it can read the body of the request where the discount data lies.
An exciting API feature we had not used yet is authentication. Our other examples were all on public APIs: they require no authentication. But in real life scenarios, most APIs you’ll use will probably require some authentication, like the Twitter API or Google Maps API, for instance. It’s a standard “pattern” in the API world. Our customers wouldn’t be so happy if we were letting anyone create discounts on their shop. 😉
Authentication concepts can become burdensome quite quickly; you can read this cool post to better master the subject.
For our purpose, we pass the API key directly in the request and hooray the server recognizes us! It can then execute the request scoped only to our account.
If you want to test our little app, you can run npm install -g
in the project directory and simply run discounts x
to create discounts x number of times. You can go in your Snipcart dashboard (forever free accounts in Test mode) and see for yourself that the discounts have been created properly. You can imagine how powerful this can be: if you had to create 200 of those discounts this would require wayyy too much time to do this via our UI. When you’re familiar with how APIs work, however, this can be done in a matter of minutes. The code is also reusable, so if you need to do so the same thing a month from now, well, you’re already all set!
If you have specific API use cases you’d like us to cover, write them in the comments. We’ll consider adding them to our content roadmap! 🙂
I genuinely hope this primer helped you better understand what APIs are, and how you can leverage them in your development workflow.
A few takeaways:
Now, our last example isn’t really “production” ready, but it gives you a good idea of how things could look like for a real life use case. There are a lot of things we could have improved, such as letting the user choose the discount type, etc. We could also use the codes created to send them via Email directly in the same function instead of merely logging them. If we had advanced needs for our discounts tools, we could have written an API ourselves. From there we could even create a UI that interacts with our API if we wanted to. Anyway, I hope you get the idea now.! 🙂
So what’s the next step? Like almost anything in the development sphere, you’ll need to code, code, code, and code some more! That’s how you’ll familiarize yourself API management & integration. After a while, you’ll become efficient with them.
And that’s where the real fun begins.
If you’ve enjoyed this post, please take a second to share it on Twitter. Got comments, questions? Hit the section below!
AI-driven updates, curated by humans and hand-edited for the Prototypr community